I’m going to create a Jenkins to enable CI/CD Pipelines.
Create a VM
Create a Linux VM with the following specifications.

Add an inbound security rule that allows access to your Jenkins VM on TCP port 8080.
Install Docker and Jenkins
sudo apt-get -y update && sudo apt-get upgrade -y
Download and add Docker CE GPG key with the following command:
wget https://download.docker.com/linux/ubuntu/gpg
apt-key add gpg
Next, add the Docker CE repository to APT with the following command:
nano /etc/apt/sources.list.d/docker.list
deb [arch=amd64] https://download.docker.com/linux/ubuntu xenial stable

Save and close the file, then update the repository.
sudo apt-get update -y
sudo apt-get install docker-ce -y
sudo systemctl status docker
Create Docker Volume for Data and Log
docker volume create jenkins-data
docker volume create jenkins-log
docker volume ls

Install Jenkins with Docker
mkdir docker
nano docker/dockerfile
Add the following lines:
FROM jenkins/jenkins
LABEL maintainer="hitjethva@gmail.com"
USER root
RUN mkdir /var/log/jenkins
RUN mkdir /var/cache/jenkins
RUN chown -R jenkins:jenkins /var/log/jenkins
RUN chown -R jenkins:jenkins /var/cache/jenkins
USER jenkins
ENV JAVA_OPTS="-Xmx4096m"
ENV JENKINS_OPTS="--handlerCountMax=300 --logfile=/var/log/jenkins/jenkins.log
--webroot=/var/cache/jenkins/war"
Note: When setting up the dockerfile for your Jenkins image, set the Java memory below 8192
cd docker
docker build -t myjenkins .
Run Jenkins Container with Data and Log Volume
docker run -p 8080:8080 -p 50000:50000 --name=jenkins-master --mount source=jenkins-log,target=/var/log/jenkins --mount source=jenkins-data,target=/var/jenkins_home -d myjenkins

Don’t forget to add inbound rule TCP port 8080.
docker exec jenkins-master tail -f /var/log/jenkins/jenkins.log
# If you need more lines, add -n option
docker exec jenkins-master tail -n 20 -f /var/log/jenkins/jenkins.log
you should see the password to proceed to installation:
b437ba21655a44cda66a75b8fbddf5b8
This may also be found at: /var/jenkins_home/secrets/initialAdminPassword
*************************************************************
*************************************************************
*************************************************************
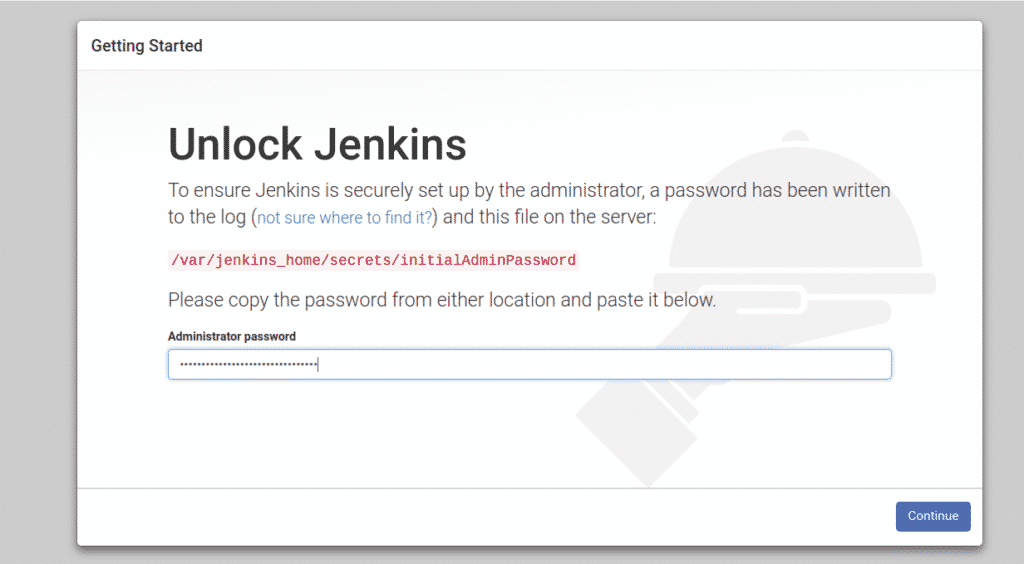
Provide your administrator password and click on the Continue button. You should see the following page:

Now, click on the “Install suggested plugins” to install the required plugins. Once the installation has been finished. You should see the following page

Now, provide your admin username, password and full name then click on the Save and Finish button. You should see the following page:

Now, just click on the Save and Finish button. Once the setup completed successfully, you should see the following page:

Troubleshooting
dial unix /var/run/docker.sock: connect: permission denied
If you get permission denied while trying to connect to the Docker daemon, you need to create the docker group and add your user.
To create the docker group and add your user:
sudo groupadd docker
sudo usermod -aG docker ${USER}
newgrp docker
docker run hello-world